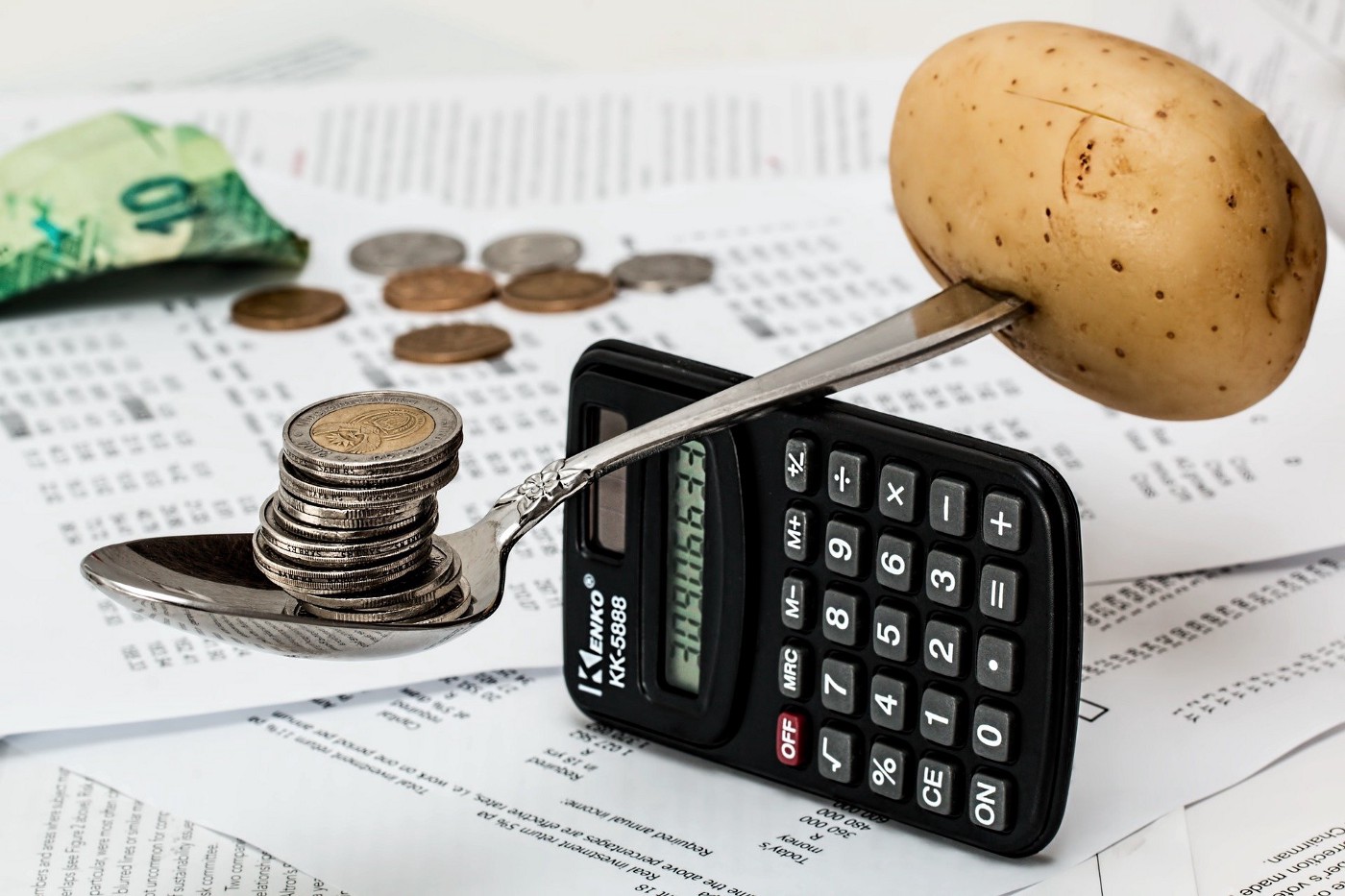
We’ll start by creating a structure that holds the data for a single trade.
A trade is made up of an amount
, a price
, and a value
. All of the trades are stored in the TradesModel
, which will make all of the calculations necessary for DCA (dollar-cost averaging).
The didSet
property observer is used so that the trades
array is given a new empty trade when the user wants to increase the number of trades displayed.
This keeps the size of the array in line with the number we expect to see on the screen, eliminating the possibility of index out-of-range errors.
The interface will take the form of a table with three columns: amount, price, and value. These appropriately align with the properties of a trade, each of which will be set using custom TextField
which will be called NumberTextField
.
Before we get started with that, we need to create a simple header row that will give us a title for each of our columns. There would be no other way to distinguish the amount from the price or value if we did not make it obvious which one was which.
You might notice that the entire HStack above has the disabled
modifier set to true
. This gives each TextField
the same appearance as others in the app, but the user cannot edit these headers to whatever they want.
The NumberTextField
bridges the gap between a TextField
, which uses a String
for input, and a Double
, which is the format we want our data to be in.
The TextField
has a decimal keypad, so the conventional alphanumeric keyboard will not be displayed. This reduces the number of possible characters dramatically, but there still isn’t anything stopping the user from inputting multiple dots. This would not convert successfully to a Double
, so we don’t want this to be possible.
An extension of String
provides a computed property that allows you to easily check whether there are multiple dots, and this is stored in the valid
property.
When the input is invalid, the TextField
will display the input as red and will not attempt to convert the value to a Double
. If it is valid, the value will be set and sent back to the Binding
.
TotalRowView
calculates the sum of all trade amounts so that you can see how much you own. The cost total is also calculated and shown here. Between them is the dollar cost average, which is calculated by dividing the total value by the total amount. Each of these values is displayed as a constant string in a TextField
that cannot be edited, so that they have the same style as the rest of the app.
TradeQuantityView
allows you to control how many rows there are. As each row represents a trade, you will want to add new trades as you make them. This row is simply a Stepper
that displays the number of trades.
Unfortunately Stepper
requires a closed range of possible values, so a maximum number of trades has to be hard-coded. This maximum number can be anything you want, but it is impossible to specify a range without a maximum value.
In some ways this is the most important view, as it allows you to input the data for the dollar cost average calculation to take place. When the amount or price TextField
is edited, the onChange
closure calls the setValue
function.
This calculates the value again, which is simply a multiplication of the other two numbers. The trade is then updated in the trades array, which is passed in as a @Binding
property. A check is made to ensure that the expected index exists in the array, preventing an index out of range error.
However, as updating a trade that doesn’t exist in the array is an unexpected behaviour, I have still added a fatalError
that explains why this is a failure state.
The main app is simply a combination of all the rows that have been created so far inside a SwiftUI Form
. This gives it an appearance much like the Settings app for iOS, and it will automatically fill the screen of any device and scroll when there are too many rows to fit onto the screen.
Views are only passed what they need, such as the total number of trades for TradeQuantityView
and the total amount and value for TotalRowView
.
A ForEach
is used to show one trade for every number up to the total number of trades that was selected by TradeQuantityView
, and the index is passed in so that the array can be updated when changes are made.
Here’s how it should look: