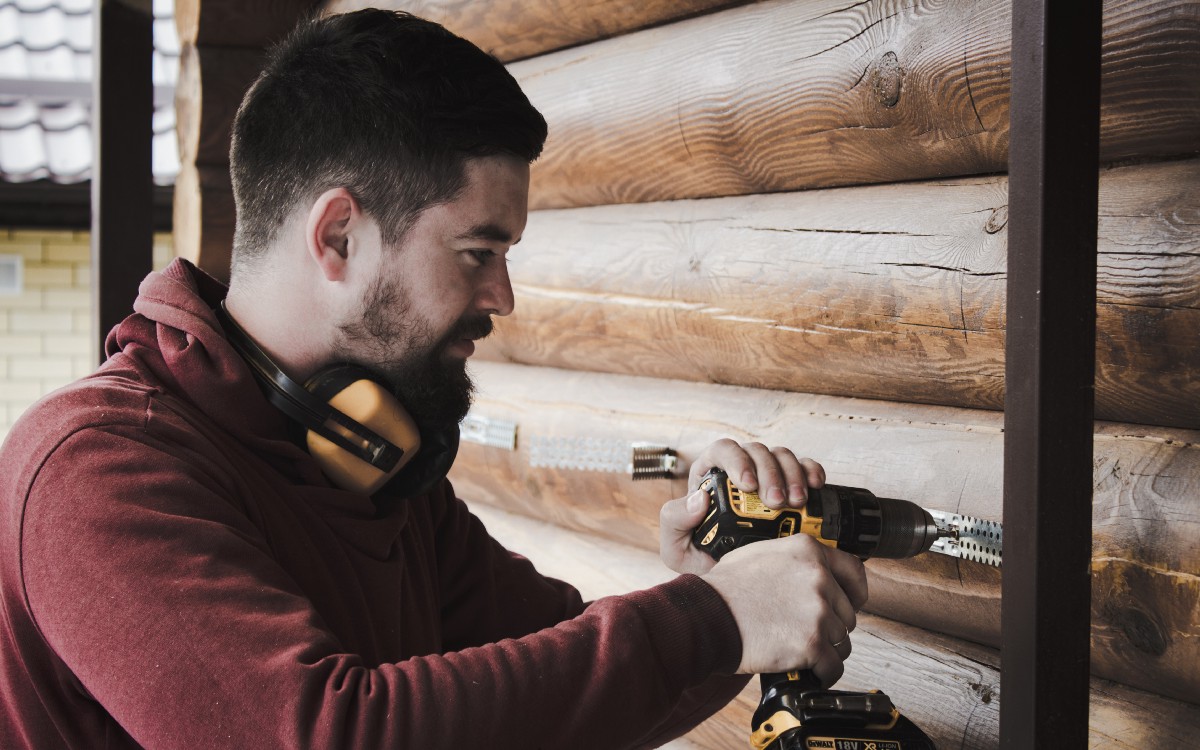
Let’s start by making a new service for users and miners. It will be a copy of the other two services for the blockchain and nodes, but we’ll put in a new controller, service, and models. Because miners are users (i.e. they need an address to include in transactions), we’ll start off with users. Let’s make an endpoint to add, list, and get information on a user. I’m going to use basic authentication for the list
and get
endpoints. The add
endpoint will be open, as anyone should be able to create a user.
While I want to demonstrate some level of authentication, I don’t want to get bogged down in the details of a true authentication system. So to simulate checking, the username and password must be the same and the special user for listing all users must be named admin. I will also create the admin user immediately if one doesn’t exist on startup.
Here’s what I have for a controller:
The service is pretty simple:
Use the application.yaml
to set the parameters for MongoDB:
Now you can use the docker-compose.yaml
file to spin up MongoDB and test out the new endpoints. You should be able to add and list users to the system. Just remember to use basic authentication. It’s important to remember that the basic authentication uses the name of the user, whereas the endpoint uses the ID for more efficient searching.
What else does a user have besides an ID and name? In a cryptocurrency system, we think of a user as having a wallet. The wallet is an address and a private/public key pair. In our system, we’re going to fold those together so a user can only have one “wallet” (i.e. an address and private/public key pair). With that, the user can create transactions. We can’t use the ID as the address because each user is attached to a node and the ID isn’t unique across nodes. So we’ll use a UUID for the address. Then it’s just a matter of creating the private/public key pair. Here’s the updated UserService.addUser
:
Because we don’t want to ever expose the privateKey
, we will add this line into any chain in the UserController
that would return a user or list of users:
.doOnNext(u -> u.setPrivateKey(null));
Now we’re ready to create a transaction on behalf of a user.
We have a rudimentary transaction creation endpoint in the blockchain service, so we’re going to remove that. We’re going to keep the add transaction endpoint and we can call that from our new endpoint in the user service. The new endpoint in the controller looks like this:
The new method in the UserService
class looks like this:
You can see that we take the private key that’s encoded in the database and use it to sign the input contract. Just like we created the withHash
method, we created the withSignature
method because the signature has to be calculated after the data is applied. We don’t bother to check the output address(es), as there’s no single source of truth for addresses. There are some specific formats for addresses used by some cryptocurrencies to help prevent giving coins away to non-existent addresses, but there’s no way to ensure that you don’t send your coins to the proverbial bit-bucket.
- admin
- All
- Authentication
- blockchain
- call
- checking
- Coins
- contract
- controller
- Creating
- cryptocurrencies
- cryptocurrency
- data
- Database
- Endpoint
- Giving
- How
- How To
- HTTPS
- ia
- information
- IP
- IT
- Java
- Key
- Level
- Line
- List
- listing
- Making
- medium
- Miners
- MongoDB
- nodes
- open
- Other
- Password
- private
- Private Key
- Services
- set
- Simple
- So
- Spin
- start
- startup
- system
- test
- transaction
- Transactions
- users
- Wallet